在 JavaScript 中,可以使用多种方法来检测一个变量是否是 String
类型。以下是几种常见的方法:
使用
typeof
运算符:typeof
运算符是检测变量类型最简单的方法。对于字符串类型,它会返回'string'
。let variable = "Hello, World!"; if (typeof variable === 'string') { console.log(variable + " is a string."); } else { console.log(variable + " is not a string."); }
使用
instanceof
运算符: 虽然String
对象在 JavaScript 中不常用(因为原始字符串和String
对象是不同的),但instanceof
运算符可以检测一个变量是否是String
对象的实例。然而,对于原始字符串类型,这种方法不适用。let primitiveString = "Hello, World!"; let stringObject = new String("Hello, World!"); console.log(primitiveString instanceof String); // false console.log(stringObject instanceof String); // true
由于原始字符串和
String
对象是不同的,通常不推荐使用instanceof
来检测字符串类型。使用
Object.prototype.toString.call
方法: 这是一个更可靠的方法,因为即使变量被包装为对象(如通过new String()
),它仍然可以正确检测类型。let variable = "Hello, World!"; if (Object.prototype.toString.call(variable) === '[object String]') { console.log(variable + " is a string."); } else { console.log(variable + " is not a string."); }
使用构造函数检查(不推荐): 可以通过检查变量的构造函数是否是
String
来判断类型,但这种方法不如typeof
安全,因为构造函数可以被更改。let variable = "Hello, World!"; if (variable.constructor === String) { console.log(variable + " is a string."); } else { console.log(variable + " is not a string."); }
总结:
推荐使用 typeof
运算符来检测字符串类型,因为它简单且高效。其他方法在某些特定情况下可能有用,但通常 typeof
是最常用和推荐的方法。
学在每日,进无止境!更多精彩内容请关注微信公众号。
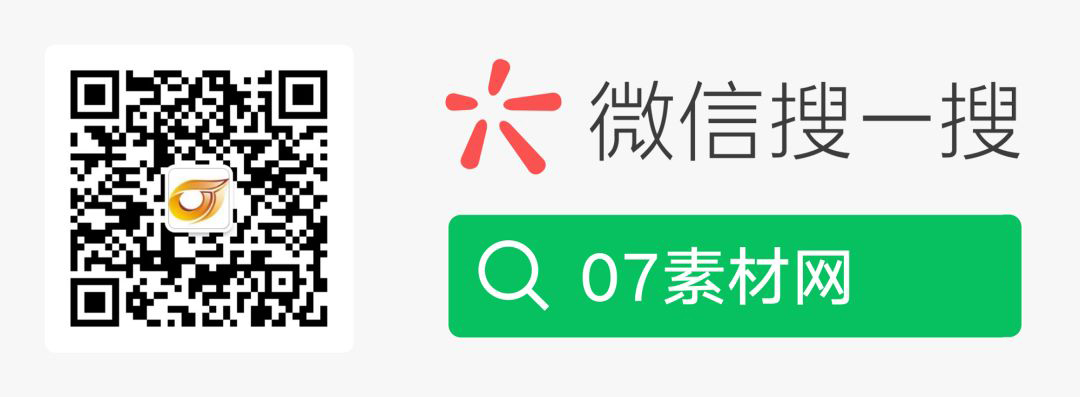
原文出处:
内容由AI生成仅供参考,请勿使用于商业用途。如若转载请注明原文及出处。
出处地址:http://www.07sucai.com/tech/380.html
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。